What is CORS?
CORS (Cross-Origin Resource Sharing) is a method that uses extra HTTP headers to notify browsers to allow a web application operating on one origin to access resources from another origin.
Why does the browser need this mechanism?
The security policy known as the “Same-origin policy” is implemented by most browsers, and it controls how information loaded from one origin interacts with resources from another origin. I won’t go into depth on why we need the same-origin policy; nevertheless, this is a highly interesting thread that discusses its utility. CORS allows the same-origin policy to be relaxed. Browsers provide us with CORS to regulate communication between apps running at different origins, rather than completely preventing it.
When we talk about CORS, we’re talking about two separate programmes attempting to communicate with one another, one of which is running in a browser.
How does it work?
When a client application running at origin A tries to access resources from a server application running at origin B, the browser will block communication between the two applications unless some specific CORS HTTP headers are present in the request and response, in which case a CORS error will appear in the browser console.
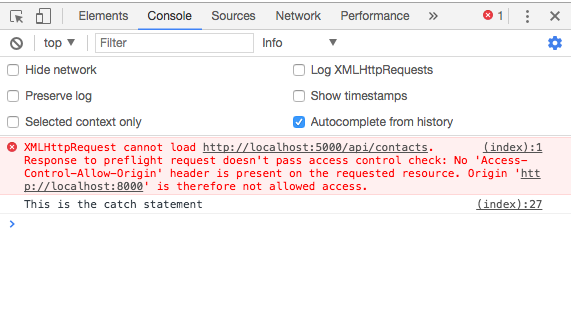
Because the browser adds the CORS headers to the request, whether a client has access to the resource or not is largely determined by the server’s CORS response headers.
A preflight request would be automatically sent by the browser for some types of access requests that could potentially cause side-effects on the server, such as a POST request to mutate data in the server, and the server would then approve or disapprove receiving the actual request via CORS HTTP headers in response to the preflight request. What exactly are CORS HTTP headers?
CORS HTTP Headers
They can be grouped into two, the request headers i.e headers in the request from the client and the Response Headers i.e headers in the response from the server.
Request Headers(All these headers are set automatically by the browser)
Origin
: This specifies the origin of the client applicationAccess-Control-Request-Method
: This is added during a preflight request to let the server know what the HTTP method the actual request intends to send.Access-Control-Request-Headers
: This is added during a preflight request to let the server know what HTTP headers the actual request intends to send.
Response Headers
Access-Control-Allow-Origin
: This specifies the origin the server wants to allow to access a resource. If this does not match theOrigin
header of the client request, the client requesting the resource would experience CORS errors.Access-Control-Allow-Headers
: This specifies the set of headers that can be present in the client request. If this does not match theAccess-Control-Request-Headers
header of the client request, the client requesting the resource would experience CORS errors.Access-Control-Allow-Methods
: This specifies the set of methods that can be used for the client request. If this does not match theAccess-Control-Request-Method
header of the client request, the client requesting the resource would experience CORS errors.Access-Control-Expose-Headers
: This specifies the set of headers from the server response that the browser can expose to the client application.Access-Control-Max-Age
: This specifies how long the result of a preflight request can be cached.Access-Control-Allow-Credentials
: For the preflight request, this indicates whether or not the actual request can make use of credentials. For CORS requests that are not preflight-ed but makes use of credentials(e.g browser cookie), this indicates whether or not the browser should allow the client app to access the response from the server.
Handling CORS
Handling CORS is actually rather straightforward, and it’s virtually always something that has to be handled by the server application.
When replying to HTTP requests in your server app, you may use the CORS response headers to tell the client app whether or not it can access a resource. Access-Control-Allow-Origin may be used to indicate which origin the client app must request from, Access-Control-Allow-Headers can be used to specify which headers the client app can send, Access-Control-Allow-Method can be used to specify which HTTP method(s) the client app can use, and so on.
There are several methods available that make managing CORS in server applications easier, and here are a few server setups that may be used.
CORS on Apache
To add the CORS authorization to the header using Apache, simply add the following line inside either the <Directory>
, <Location>
, <Files>
or <VirtualHost>
sections of your server config (usually located in a *.conf file, such as httpd.conf or apache.conf), or within a .htaccess
file:
<IfModule mod_headers.c>
Header set Access-Control-Allow-Origin "*"
</IfModule>
To ensure that your changes are correct, it is strongly recommended that you use
apachectl -t
to check your configuration changes for errors. After this passes, you may need to reload Apache to make sure your changes are applied by running the command
sudo service apache2 reload
or
apachectl -k graceful
Altering headers requires the use of mod_headers. Mod_headers is enabled by default in Apache, however, you may want to ensure it’s enabled by run
a2enmod headers
Note: you can also use add
rather than set
, but be aware that add
can add the header multiple times, so it’s likely safer to use the set.
CORS on ASP.NET
If you don’t have access to configure IIS, you can still add the header through ASP.NET by adding the following line to your source pages:
Response.AppendHeader("Access-Control-Allow-Origin", "*");
Note: this approach is compatible with IIS6, IIS7 Classic Mode, and IIS7 Integrated Mode.
ASP.NET Web API
ASP.NET Web API 2 supports CORS.
To enable CORS support, add Microsoft.AspNet.WebApi.Cors NuGet package to your project.
Add this code to your configuration:
public static void Register(HttpConfiguration config)
{
// New code
config.EnableCors();
}
To enable cross-origin requests, add the [EnableCors] attribute to your Web API controller or controller method:
[EnableCors(origins: "http://example.com", headers: "*", methods: "*")]
public class TestController : ApiController
{
// Controller methods not shown...
}
Enabling Globally
The method described above can also be used to enable CORS across the API without annotating each controller:
public static void Register(HttpConfiguration config)
{
var corsAttr = new EnableCorsAttribute("http://example.com", "*", "*");
config.EnableCors(corsAttr);
}
For more information, see the official Web API documentation.
Libraries
- The open source Thinktecture.IdentityModel security library contains a full-featured CORS implementation. Here is the announcement page with some samples. Here is the source for .NET 4.5 and for .NET 4.0. Both are packaged into a Nuget package downloadable from Visual Studio.
CORS on AWS API Gateway
Amazon API Gateway adds support for CORS enabling through a simple button in the API Gateway console. Unfortunately, that button has a partial behaviour, thus setting CORS correctly only for 200 answer (so not other HTTP status codes) and ignoring JQuery header support. The best solution considered so far is about avoiding using the CORS button and setting configurations manually. This can be achieved in a couple of steps:
- Log into API Gateway console
- Create all the REST resources that needs to be exposed with their methods before setting up CORS (if new resources/methods are created after enabling CORS, these steps must be repeated)
- Select a resource
- Add OPTIONS method, choose as integration type “mock”
- For each Method of a resource
- Go to Response Method
- Add all the response method that should be supported (i.e. 200, 500, etc.)
- For each response code set Response Headers to
- X-Requested-With
- Access-Control-Allow-Headers
- Access-Control-Allow-Origin
- Access-Control-Allow-Methods
- Go to Integration Response, select one of the created response codes, then Header Mappings
- Insert default values for headers
example:- X-Requested-With: ‘*’
- Access-Control-Allow-Headers: ‘Content-Type,X-Amz-Date,Authorization,X-Api-Key,x-requested-with’
- Access-Control-Allow-Origin: ‘*’
- Access-Control-Allow-Methods: ‘POST,GET,OPTIONS’
- Deploy the API to a stage
- Check using http://client.cors-api.appspot.com/client that CORS requests have been successfully enabled
CORS on ExpressJS
In your ExpressJS app on node.js, do the following with your routes:
app.use(function(req, res, next) {
res.header("Access-Control-Allow-Origin", "YOUR-DOMAIN.TLD"); // update to match the domain you will make the request from
res.header("Access-Control-Allow-Headers", "Origin, X-Requested-With, Content-Type, Accept");
next();
});
app.get('/', function(req, res, next) {
// Handle the get for this route
});
app.post('/', function(req, res, next) {
// Handle the post for this route
});
CORS on IIS7
Adding required headers for underlying CORS handling
For Microsoft IIS7, merge this into the web.config
file at the root of your application or site:
<?xml version="1.0" encoding="utf-8"?>
<configuration>
<system.webServer>
<httpProtocol>
<customHeaders>
<add name="Access-Control-Allow-Origin" value="*" />
</customHeaders>
</httpProtocol>
</system.webServer>
</configuration>
If you don’t have a web.config
file already, or don’t know what one is, just create a new file called web.config
containing the snippet above.
CORS on Spring Boot Applications in Kotlin
The WebFilter in the Kotlin code block below enables CORS on Spring Boot applications using the WebFlux starter.
import org.springframework.http.HttpMethod
import org.springframework.http.HttpStatus
import org.springframework.stereotype.Component
import org.springframework.web.server.ServerWebExchange
import org.springframework.web.server.WebFilter
import org.springframework.web.server.WebFilterChain
import reactor.core.publisher.Mono
@Component
class CorsFilter : WebFilter {
override fun filter(ctx: ServerWebExchange?, chain: WebFilterChain?): Mono<Void> {
if (ctx != null) {
ctx.response.headers.add("Access-Control-Allow-Origin", "*")
ctx.response.headers.add("Access-Control-Allow-Methods", "GET, PUT, POST, DELETE, OPTIONS")
ctx.response.headers.add("Access-Control-Allow-Headers", "DNT,X-CustomHeader,Keep-Alive,User-Agent,X-Requested-With,If-Modified-Since,Cache-Control,Content-Type,Content-Range,Range")
if (ctx.request.method == HttpMethod.OPTIONS) {
ctx.response.headers.add("Access-Control-Max-Age", "1728000")
ctx.response.statusCode = HttpStatus.NO_CONTENT
return Mono.empty()
} else {
ctx.response.headers.add("Access-Control-Expose-Headers", "DNT,X-CustomHeader,Keep-Alive,User-Agent,X-Requested-With,If-Modified-Since,Cache-Control,Content-Type,Content-Range,Range")
return chain?.filter(ctx) ?: Mono.empty()
}
} else {
return chain?.filter(ctx) ?: Mono.empty()
}
}
}
CORS on Nginx
The following Nginx configuration enables CORS, with support for preflight requests.
#
# Wide-open CORS config for nginx
#
location / {
if ($request_method = 'OPTIONS') {
add_header 'Access-Control-Allow-Origin' '*';
add_header 'Access-Control-Allow-Methods' 'GET, POST, OPTIONS';
#
# Custom headers and headers various browsers *should* be OK with but aren't
#
add_header 'Access-Control-Allow-Headers' 'DNT,User-Agent,X-Requested-With,If-Modified-Since,Cache-Control,Content-Type,Range';
#
# Tell client that this pre-flight info is valid for 20 days
#
add_header 'Access-Control-Max-Age' 1728000;
add_header 'Content-Type' 'text/plain; charset=utf-8';
add_header 'Content-Length' 0;
return 204;
}
if ($request_method = 'POST') {
add_header 'Access-Control-Allow-Origin' '*' always;
add_header 'Access-Control-Allow-Methods' 'GET, POST, OPTIONS' always;
add_header 'Access-Control-Allow-Headers' 'DNT,User-Agent,X-Requested-With,If-Modified-Since,Cache-Control,Content-Type,Range' always;
add_header 'Access-Control-Expose-Headers' 'Content-Length,Content-Range' always;
}
if ($request_method = 'GET') {
add_header 'Access-Control-Allow-Origin' '*' always;
add_header 'Access-Control-Allow-Methods' 'GET, POST, OPTIONS' always;
add_header 'Access-Control-Allow-Headers' 'DNT,User-Agent,X-Requested-With,If-Modified-Since,Cache-Control,Content-Type,Range' always;
add_header 'Access-Control-Expose-Headers' 'Content-Length,Content-Range' always;
}
}
CORS on PHP
If you don’t have access to configure Apache, you can still send the header from a PHP script. It’s a case of adding the following to your PHP scripts:
<?php
header("Access-Control-Allow-Origin: *");
Note: as with all uses of the PHP header function, this must be before any output has been sent from the server.
CORS on Tomcat
Apache Tomcat includes support for CORS (Starting from Tomcat version 7.0.41). The full documentation can be found here: http://tomcat.apache.org/tomcat-7.0-doc/config/filter.html#CORS_Filter
Here is an example from those docs that demonstrates a minimal CORS configuration:
<filter>
<filter-name>CorsFilter</filter-name>
<filter-class>org.apache.catalina.filters.CorsFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>CorsFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>